Scope
- Use Case:
- Consider the situation where a number of orders that have been added to a job chain - either from a file order source watching incoming files, or from an external source such as JOC that adds ad hoc orders or from permanent orders. These orders should then be processed serially in a predefined sorting order.
- Solution Outline:
- A single job is added at the beginning of a job chain that will:
- suspend all incoming orders and check completeness until a predefined idle timeout has beens reached and no more orders are expected,
- sort the orders in alphabetical sequence according to the order ID and move them to the next job node in the job chain.
- A single job is added at the beginning of a job chain that will:
- References
Solution
- Download sort_orders.zip
- Extract the archive to any folder within the
./config/live
folder of your JobScheduler installation. - The archive extracts the files to a folder named
sort_orders.
- You can store the sample files in any folder as you like, the solution does not make use of specific folder or job names.
Pattern
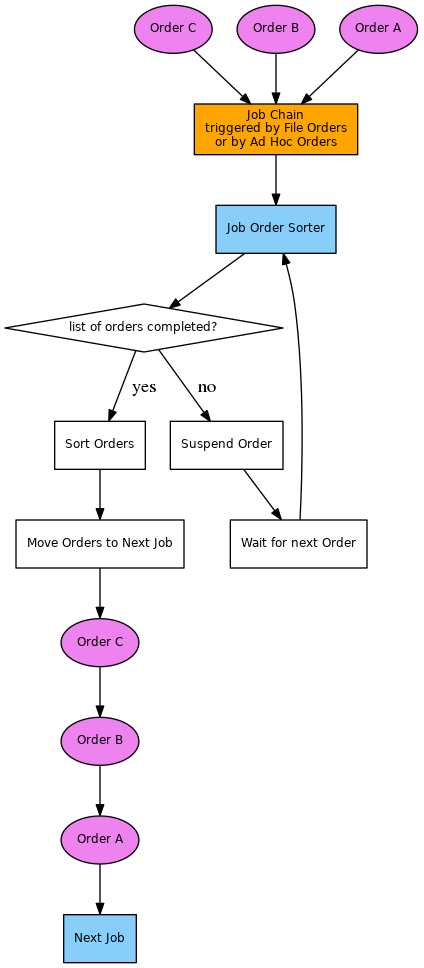
Implementation
Components
- The solution implements a job named
sorter
that can be added at the start of any job chain.- This job implements a
spooler_process()
function that suspends all incoming orders. - This job is configured for a single task and with an idle timeout attribute. This means that it will execute incoming orders sequentially.
- Having received the last available order this job will wait for the duration specified with the
idle_timeout
attribute for new orders.- The idle timeout is configured using, for example
<job idle_timeout="10">
with thesorter
job definition. - Once the idle timeout has expired this job will execute its
spooler_exit()
function and then sort and move all orders that have previously been suspended.- Sorting is done in alphabetical order.
- The orders are moved to the next job chain node that follows the
sorter
job in the job chain.
- The idle timeout is configured using, for example
- This job implements a
- The download example uses a job chain named
job_chain1
that includes the job nodes for thesorter
job and ahello
job. This job chain accepts ad hoc orders that are added by JOC and it can easily be modified to watch for incoming files and to create an order for each file. - Hint: to re-use the
sorter
job you can:- store the job in a central folder and reference the job in individual job chains.
- move the job's JavaScript code to a central location and use an appropriate
<include>
element for individual job scripts.
Usage
- Add two orders to the
job_chain1
job chain.- Use an order ID in descending alphabetical order, e.g. "cba" for the order ID of the first order and "abc" for the order ID of the second.
- Both orders will be suspended at the first node of the job chain.
- After an idle timeout of 10s both orders will be moved to the next job node in the job chain.
- This time the orders will be processed in ascending alphabetical order.