Scope
This document explains how to integrate the JobScheduler .jar archive for Java API jobs (engine-job-api) from Maven Central into a maven project.
Aditionally this document provides a small example to create a Java API job class and how to use it in a JobScheduler job.
Prerequisites
-
FEATURE AVAILABILITY STARTING FROM RELEASE 1.9.8
- Starting with this release the JobScheduler engine-job-api (jar) and the engine (pom) artifacts are available from http://mvnrepository.com/.
- In order to download the artifacts and to integrate them in Java projects it is required that Maven is installed.
Maven
The Artifacts
engine-job-api-1.9.8.jar
engine-1.9.8.pom
The release number 1.9.8
from this sample is based on the semantic versioning scheme. This sample release number is used for the examples in this article.
The Dependency Configuration
To integrate the library into your own Maven project or to download it via Maven, add the following configuration to the pom.xml
file of your project.
<dependencies>
...
<dependency>
<groupId>com.sos-berlin.jobscheduler.engine</groupId>
<artifactId>engine-job-api</artifactId>
<version>1.9.8</version>
</dependency>
...
</dependencies>
Example of a Java Job using the engine-job-api
This is a small example of a Java API Job, which demonstrates the usage of the engine-job-api
. It implements the spooler_process()
method with a simple call of spooler_log()
method.
package com.sos.berlin.example;
import sos.spooler.Job_impl;
public class SimpleJobApiExample extends Job_impl {
@Override
public boolean spooler_process() throws Exception {
spooler_log.info("Hallo world!");
return false;
}
}
The structure of the pom.xml
file from the example:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.sos-berlin</groupId>
<artifactId>hallo-world-example-job-api</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>${project.artifactId}</name>
<description>this is a simple hallo-world-example for the usage of the engine-job-api</description>
<dependencies>
<dependency>
<groupId>com.sos-berlin.jobscheduler.engine</groupId>
<artifactId>engine-job-api</artifactId>
<version>1.9.8</version>
</dependency>
</dependencies>
</project>
Example of the Maven log from the build of the example:
[INFO] Scanning for projects...
[INFO]
[INFO] Using the builder org.apache.maven.lifecycle.internal.builder.singlethreaded.SingleThreadedBuilder with a thread count of 1
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] Building hallo-world-example-job-api 0.0.1-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] Downloading: http://repo.maven.apache.org/maven2/com/sos-berlin/jobscheduler/engine/engine-job-api/1.9.8/engine-job-api-1.9.8.pom
[INFO] Downloaded: http://repo.maven.apache.org/maven2/com/sos-berlin/jobscheduler/engine/engine-job-api/1.9.8/engine-job-api-1.9.8.pom (5 KB at 5.6 KB/sec)
[INFO] Downloading: http://repo.maven.apache.org/maven2/com/sos-berlin/jobscheduler/engine/engine-job-api/1.9.8/engine-job-api-1.9.8.jar
[INFO] Downloaded: http://repo.maven.apache.org/maven2/com/sos-berlin/jobscheduler/engine/engine-job-api/1.9.8/engine-job-api-1.9.8.jar (79 KB at 108.0 KB/sec)
[INFO]
[INFO] --- maven-clean-plugin:2.5:clean (default-clean) @ hallo-world-example-job-api ---
[INFO] Deleting C:\sp\testing\test-projects\hallo-world-example-job-api\target
[INFO]
[INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ hallo-world-example-job-api ---
[INFO] skip non existing resourceDirectory C:\sp\testing\test-projects\hallo-world-example-job-api\src\main\resources
[INFO]
[INFO] --- maven-compiler-plugin:2.5.1:compile (default-compile) @ hallo-world-example-job-api ---
[INFO] Compiling 1 source file to C:\sp\testing\test-projects\hallo-world-example-job-api\target\classes
[INFO]
[INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ hallo-world-example-job-api ---
[INFO] skip non existing resourceDirectory C:\sp\testing\test-projects\hallo-world-example-job-api\src\test\resources
[INFO]
[INFO] --- maven-compiler-plugin:2.5.1:testCompile (default-testCompile) @ hallo-world-example-job-api ---
[INFO] No sources to compile
[INFO]
[INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ hallo-world-example-job-api ---
[INFO] No tests to run.
[INFO]
[INFO] --- maven-jar-plugin:2.4:jar (default-jar) @ hallo-world-example-job-api ---
[INFO] Building jar: C:\sp\testing\test-projects\hallo-world-example-job-api\target\hallo-world-example-job-api-0.0.1-SNAPSHOT.jar
[INFO]
[INFO] --- maven-install-plugin:2.4:install (default-install) @ hallo-world-example-job-api ---
[INFO] Installing C:\sp\testing\test-projects\hallo-world-example-job-api\target\hallo-world-example-job-api-0.0.1-SNAPSHOT.jar to C:\Users\Santiago\.m2\repository\com\sos-berlin\hallo-world-example-job-api\0.0.1-SNAPSHOT\hallo-world-example-job-api-0.0.1-SNAPSHOT.jar
[INFO] Installing C:\sp\testing\test-projects\hallo-world-example-job-api\pom.xml to C:\Users\Santiago\.m2\repository\com\sos-berlin\hallo-world-example-job-api\0.0.1-SNAPSHOT\hallo-world-example-job-api-0.0.1-SNAPSHOT.pom
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 3.023 s
[INFO] Finished at: 2015-12-17T15:03:31+01:00
[INFO] Final Memory: 16M/167M
[INFO] ------------------------------------------------------------------------
Using the Java Job
Copy the jar file
After you have built your Maven Project copy the resulting jar archive from the target folder of your build to the ${SCHEDULER_HOME}/lib/user_lib
folder of your JobScheduler installation.
Create a Job with JOE
To use this Java API class configure a job in JOE like this:
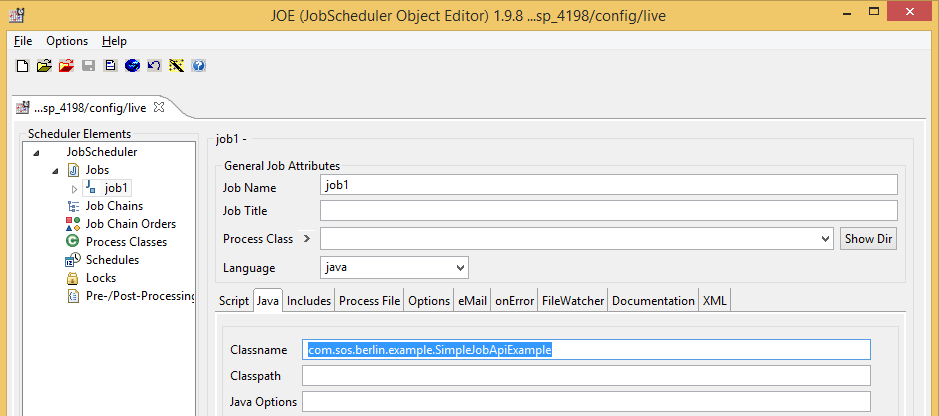
Run the Job with JOC
Run the Job in JOC and check the outcome in the Task History. The outcome should look like this:
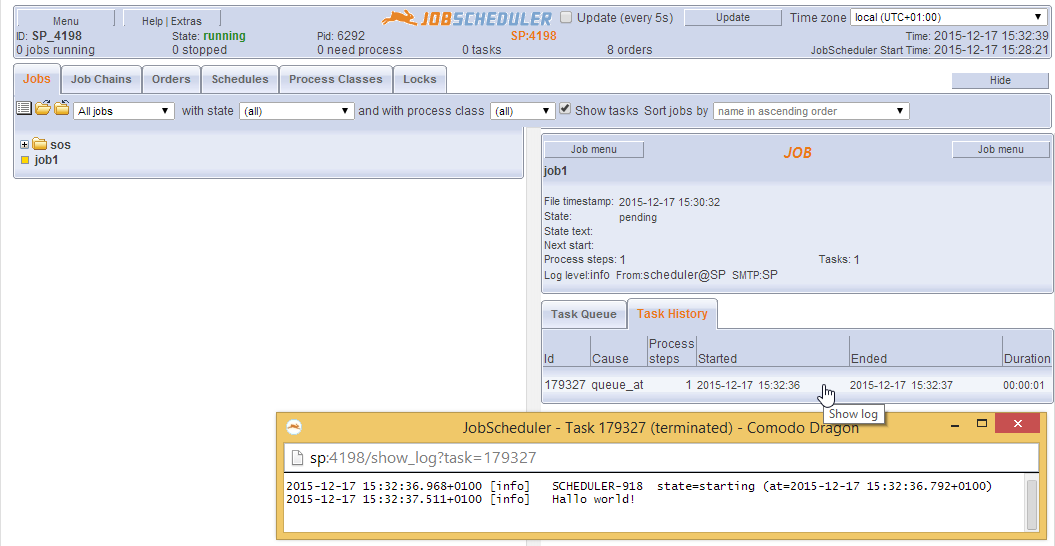
To run this example configure the job as shown above or download the example zip file from here.